Visual Studio is Microsoft's IDE (integrated development environment), and since C# is one of Microsoft's languages (and its only good one lol), we'll use VS for this tutorial.
The Community version is free to download and use without a subscription or Microsoft account. Download it here. :)
VS should come preconfigured with everything you need to start a C# project, so let's create our first project.
Creating a first project
Open up Visual Studio. The start menu should look similar to the one below. Click Create a new project.
Search "console app" on the next menu. You'll want the option with the little green C# symbol in the corner, but not the one that says .NET Framework.
.NET Framework was Microsoft's older version of .NET and C#, which only supported Windows. It's since been replaced with .NET CORE, which is cross-platform and more up-to-date.
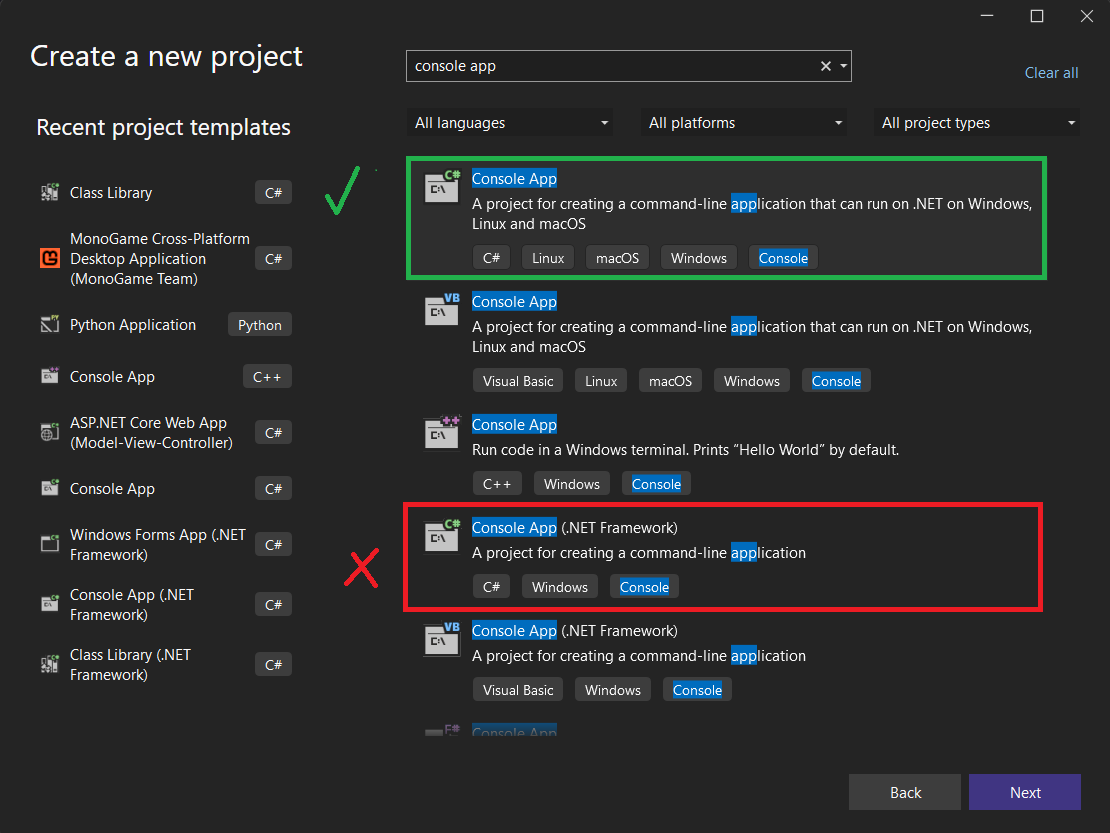
Name your project "HelloWorld". C# projects and file names are typically written in Pascal case (WhichLooksLikeThis). Your path doesn't matter much.
You're free to leave the Place solution and project in the same directory box ticked for this chapter. I tend to leave it unchecked, because I often have multiple projects per solution. A "solution" is simply a collection of one or more projects, consisting or one or more C# files, that are used to "solve" your programming problem.
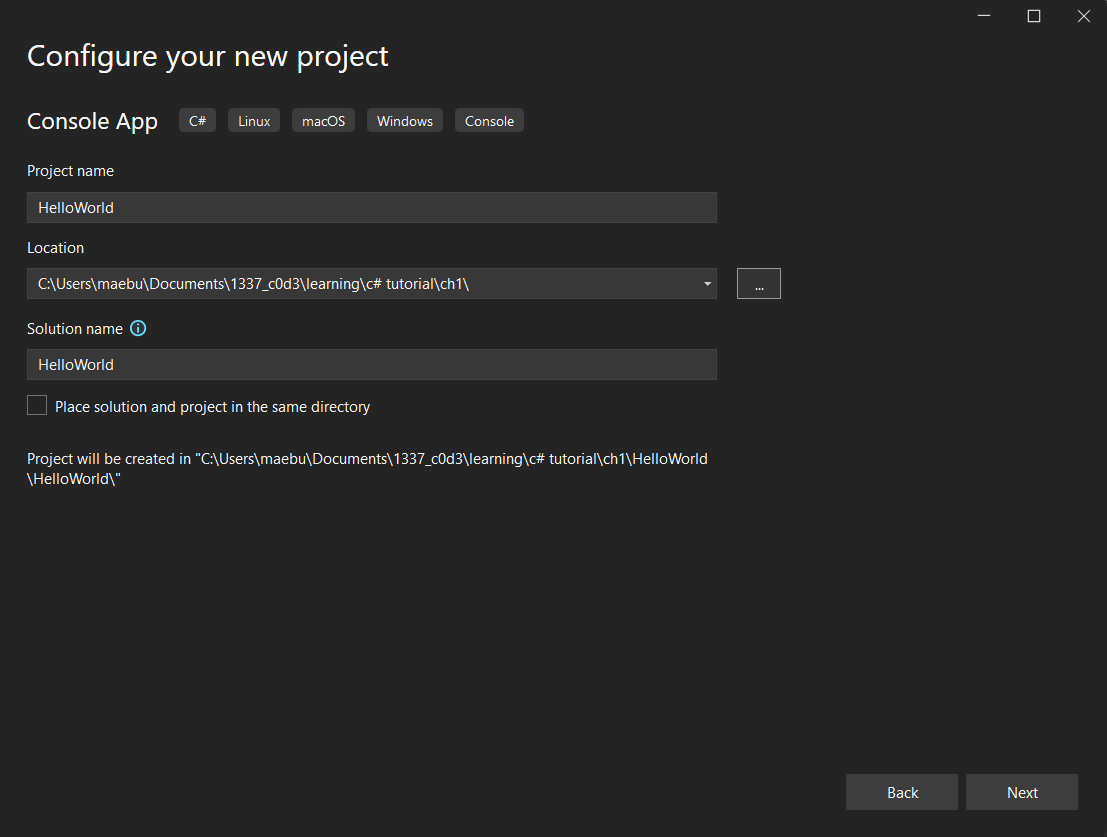
Lastly, we'll leave the framework version as .NET 6.0, since it's the current LTS version. Please check the Do not use top-level statements box, otherwise VS will hide a lot of information useful to this tutorial. :( Then click Create.
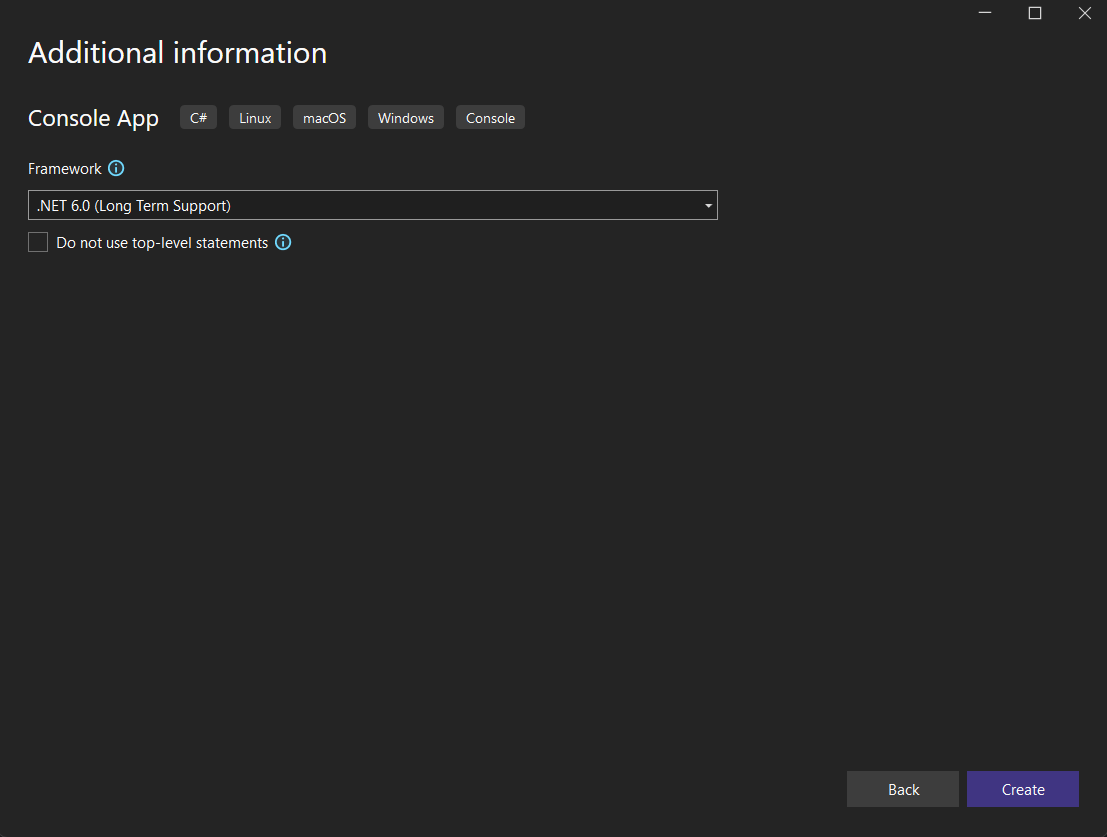
"Hello, World!"
If you get into programming and learn multiple languages, these Hello World programs will become an annoyance eventually. But for now they're useful to dissect and understand the basics. VS even starts out your fresh project with a Hello World template.
Your screen should look something like this:
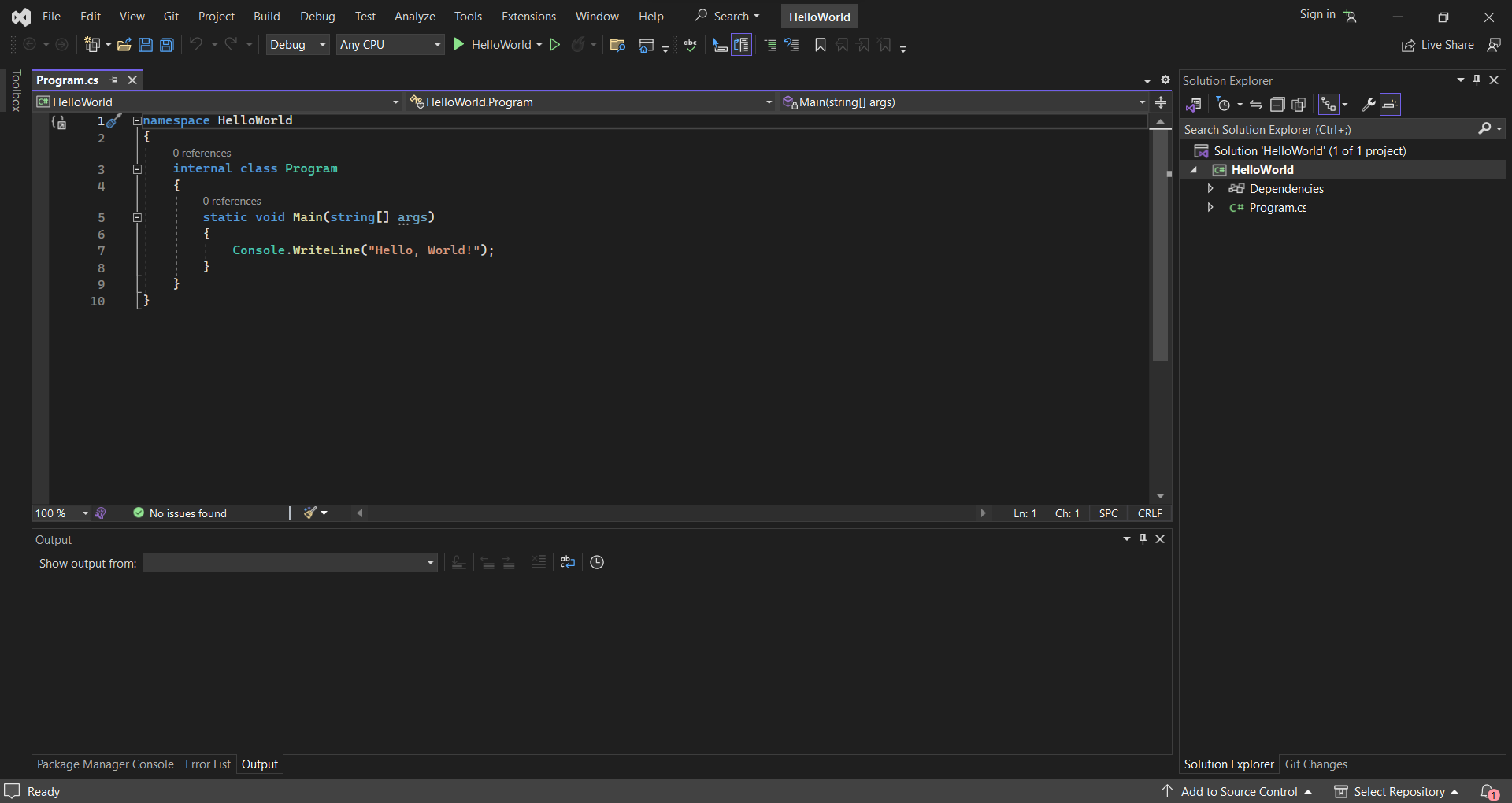
Let's dissect this beast.
namespace HelloWorld
{
internal class Program
{
static void Main(string[] args)
{
Console.WriteLine("Hello, World!");
}
}
}
Our file's namespace is a sort of named storage space for classes. Any class placed under this HelloWorld namespace can be accessed by referencing the HelloWorld namespace. This isn't super important right now. I'll go into more detail when we start creating projects with more than one file.
Like many other languages, C# applications have a "main" function. In C#, it's housed in the Program class, which is internal to ensure nothing outside of the application can access the code inside it. This main function is always the very first thing to run in our program. This will become more relevant when we start writing other functions.
I don't want to overwhelm you with jargon, so the other aspects of this Hello World application will be explained as we go through aspects of the C# language.
Lastly, our single line within the main function prints the string "Hello, World!" to the command line/console output. :)
Go ahead and click the Run button in the top toolbar to build and run this project, which is a green play button next to the name of your project. A console should open and show you the results.
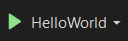
